Mobile App Dev 2021W: Tutorial 1
In this tutorial you will extend and study a simple app for temperature conversion. Please submit your answers on cuLearn by January 24, 2021.
Getting Started
First, you should configure your Xcode environment and make sure you can successfully run an automatically generated hello world program. Once that all works, move on to the exercises below.
Download Converter-1
Download Converter-1.zip and unpack it in your code directory. Open the project in Xcode. It should look similar to the hello world program you created previously, except this one has a bit more code. When you refresh the preview, it should look like the following:
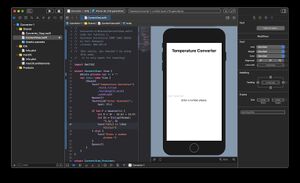
Make sure you can run the project in the simulator.
Tasks
For each task, explain what you did to accomplish the task or explain your attempts at an answer. Be sure to include references (links) to any resources that you used for each question, as well as the names of any collaborators.
Be sure to use this template when submitting answers on cuLearn. When a question asks for code, explain the changes you made and include relevant code snippets. While tutorials are graded for participation, approach them like assignments, as these are just practice assignments.
(While you may adjust text spacing and character formatting to improve readability, be sure to include all of the requested information. Also, make sure to turn in a text file, not a Word or PDF file!)
- Change the format of the output Celsius to have two fixed decimal places rather than have a general format with four significant digits.
- Add a line below the Celsius conversion that gives the temperature in Kelvin.
- Make the temperatures bold only (the rest of the text should be as it was). It is okay if there is extra spacing between the words and numbers.
- Add a miles to kilometers converter to the same screen. It should be below the existing Fahrenheit converter. The input for miles and Fahrenheit should be separate.
- What are the keywords used in the ContenView struct? What are the explicit types? What are the implicit types? There are lots, so don't worry if you can't get them all. However, try to identify as many of them as you can.
- Out of the ones you identify, which keywords and types seem mysterious?
Code
//
// Converter-1/Shared/ContentView.swift
// code for Tutorial 1,
// Carleton University COMP 1601 2021W
// by Anil Somayaji
// License: GNU GPLv3
//
// (But really, you shouldn't be using this code,
// it is only meant for teaching)
import SwiftUI
struct ContentView: View {
@State private var Fs = ""
var body: some View {
VStack{
Text("Temperature Converter")
.font(.title)
.fontWeight(.bold)
.padding()
Spacer()
TextField("Enter Farenheit", text: $Fs)
if let F = Double(Fs) {
let C = (F - 32.0) * (5/9)
let Cs = String(format: "%.4g", C)
Text("\(Fs) is \(Cs) Celsius")
} else {
Text("Enter a number please.")
}
Spacer()
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
Solutions
Solutions are now available.